Widgets
In this part of the Visual Basic GTK# programming tutorial, we will introduce some widgets.Widgets are basic building blocks of a GUI application. Over the years, several widgets became a standard in all toolkits on all OS platforms. For example a button, a check box or a scroll bar. The GTK# toolkit's philosophy is to keep the number of widgets at a minimum level. More specialized widgets are created as custom GTK# widgets.
CheckButton
CheckButton is a widget, that has two states. On and Off. The On state is visualised by a check mark. It is used to denote some boolean property.' ZetCode Mono Visual Basic GTK# tutorialWe will display a title in the titlebar of the window, depending on the state of the CheckButton.
'
' This program toggles the title of the
' window with the CheckButton widget
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Public Sub New
MyBase.New("CheckButton")
Me.InitUI
Me.SetDefaultSize(250, 150)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim cb As New CheckButton("Show title")
cb.Active = True
AddHandler cb.Toggled, AddressOf Me.OnToggle
Dim fix As New Fixed
fix.Put(cb, 50, 50)
Me.Add(fix)
End Sub
Sub OnToggle(ByVal sender As Object, ByVal args As EventArgs)
If sender.Active
Me.Title = "CheckButton"
Else
Title = " "
End If
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim cb As New CheckButton("Show title")CheckButton widget is created.
cb.Active = TrueThe title is visible by default, so we check the check button by default.
If sender.ActiveDepending on the Active property of the CheckButton, we show or hide the title of the window.
Me.Title = "CheckButton"
Else
Title = " "
End If
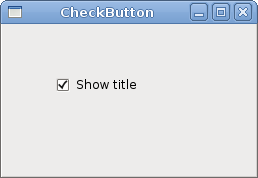
Figure: CheckButton
Label
The Label widget shows text.' ZetCode Mono Visual Basic GTK# tutorialThe code example shows some lyrics on the window.
' This program shows lyrics on
' the window in a label widget
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Dim text As String = "Meet you downstairs in the bar and heard" & vbNewLine & _
"your rolled up sleeves and your skull t-shirt" & vbNewLine & _
"You say why did you do it with him today?" & vbNewLine & _
"and sniff me out like I was Tanqueray" & vbNewLine & _
"" & vbNewLine & _
"cause you're my fella, my guy" & vbNewLine & _
"hand me your stella and fly" & vbNewLine & _
"by the time I'm out the door" & vbNewLine & _
"you tear men down like Roger Moore" & vbNewLine & _
"" & vbNewLine & _
"I cheated myself" & vbNewLine & _
"like I knew I would" & vbNewLine & _
"I told ya, I was trouble" & vbNewLine & _
"you know that I'm no good"
Public Sub New
MyBase.New("You know I'm No Good")
Me.InitUI
Me.SetPosition(WindowPosition.Center)
Me.BorderWidth = 10
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim lyrics As New Label(text)
Me.Add(lyrics)
End Sub
Sub OnDelete(ByVal sender As Object, ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim text As String = "Meet you downstairs in the bar and heard" & vbNewLine & _We define a multi line text. Unlike in C#, Python or Ruby, there is no simple construct to create a multi line text in Visual Basic language. To create a multi line text in Visual Basic, we use the vbNewLineprint constant, the + concatenation character and the _ line termination character.
"your rolled up sleeves and your skull t-shirt" & vbNewLine & _
...
Me.BorderWidth = 10The Label is surrounded by some empty space.
Dim lyrics As New Label(text)The Label widget is created and added to the window.
Me.Add(lyrics)
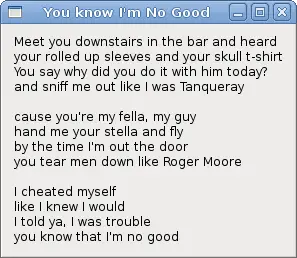
Figure: Label Widget
Entry
The Entry is a single line text entry field. This widget is used to enter textual data.' ZetCode Mono Visual Basic GTK# tutorialThis example shows an entry widget and a label. The text that we key in the entry is displayed immediately in the label widget.
'
' This program demonstrates the
' Entry widget. Text entered in the Entry
' widget is shown in a Label widget.
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Dim label As Label
Public Sub New
MyBase.New("Entry")
Me.InitUI
Me.SetDefaultSize(250, 150)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim fixed As New Fixed
label = New Label("...")
fixed.put(label, 60, 40)
Dim entry As New Entry
fixed.put(entry, 60, 100)
AddHandler entry.Changed, AddressOf Me.OnTextChanged
Me.Add(fixed)
End Sub
Sub OnTextChanged(ByVal sender As Object, _
ByVal args As EventArgs)
label.Text = sender.Text
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim entry As New EntryEntry widget is created.
AddHandler entry.Changed, AddressOf Me.OnTextChangedIf the text in the Entry widget is changed, we call the OnTextChanged method.
Sub OnTextChanged(ByVal sender As Object, _We get the text from the Entry widget and set it to the label.
ByVal args As EventArgs)
label.Text = sender.Text
End Sub
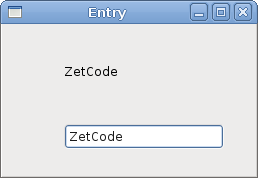
Figure: Entry Widget
ToggleButton
ToggleButton is a button that has two states. Pressed and not pressed. You toggle between these two states by clicking on it. There are situations where this functionality fits well.' ZetCode Mono Visual Basic GTK# tutorialIn our example, we show three toggle buttons and a DrawingArea. We set the background color of the area to black. The togglebuttons will toggle the red, green and blue parts of the color value. The background color will depend on which togglebuttons we have pressed.
'
' This program uses toggle buttons to
' change the background color of
' a widget.
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Dim darea As DrawingArea
Dim color As Gdk.Color
Public Sub New
MyBase.New("Togggle buttons")
Me.InitUI
Me.SetDefaultSize(350, 240)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
color = New Gdk.Color(0, 0, 0)
Dim redb As New ToggleButton("Red")
redb.SetSizeRequest(80, 35)
AddHandler redb.Toggled, AddressOf Me.OnToggled
Dim greenb As New ToggleButton("Green")
greenb.SetSizeRequest(80, 35)
AddHandler greenb.Toggled, AddressOf Me.OnToggled
Dim blueb As New ToggleButton("Blue")
blueb.SetSizeRequest(80, 35)
AddHandler blueb.Toggled, AddressOf Me.OnToggled
darea = New DrawingArea
darea.SetSizeRequest(150, 150)
darea.ModifyBg(StateType.Normal, color)
Dim fixed As New Fixed
fixed.Put(redb, 30, 30)
fixed.Put(greenb, 30, 80)
fixed.Put(blueb, 30, 130)
fixed.Put(darea, 150, 30)
Me.Add(fixed)
End Sub
Private Sub OnToggled(ByVal sender As Object, _
ByVal args As EventArgs)
Dim red As Integer = color.Red
Dim green As Integer = color.Green
Dim blue As Integer = color.Blue
If sender.Label.Equals("Red")
If sender.Active
color.Red = 65535
Else
color.Red = 0
End If
End If
If sender.Label.Equals("Green")
If sender.Active
color.Green = 65535
Else
color.Green = 0
End If
End If
If sender.Label.Equals("Blue")
If sender.Active
color.Blue = 65535
Else
color.Blue = 0
End If
End If
darea.ModifyBg(StateType.Normal, color)
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
color = New Gdk.Color(0, 0, 0)This is the color value that is going to be updated with the toggle buttons.
Dim redb As New ToggleButton("Red")The ToggleButton widget is created. We set it's size to 80x35 pixels. Each of the toggle buttons has the same handler method.
redb.SetSizeRequest(80, 35)
AddHandler redb.Toggled, AddressOf Me.OnToggled
darea = New DrawingAreaThe DrawingArea widget is the widget, that displays the color, mixed by the toggle buttons. At start, it shows black color.
darea.SetSizeRequest(150, 150)
darea.ModifyBg(StateType.Normal, color)
If sender.Label.Equals("Red")We update the red part of the color according to the value of the Active property.
If sender.Active
color.Red = 65535
Else
color.Red = 0
End If
End If
darea.ModifyBg(StateType.Normal, color)We update the color of the DrawingArea widget.
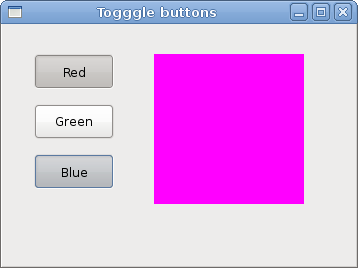
Figure: ToggleButton widget
ComboBox
ComboBox is a widget that allows the user to choose from a list of options.' ZetCode Mono Visual Basic GTK# tutorialThe example shows a combo box and a label. The combo box has a list of six options. These are the names of Linux Distros. The label widget shows the selected option from the combo box.
'
' In this program, we use the ComboBox
' widget to select an option.
' The selected option is shown in the
' Label widget
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Dim lbl As Label
Public Sub New
MyBase.New("ComboBox")
Me.InitUI
Me.SetDefaultSize(350, 240)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim distros() As String = New String() { _
"Ubuntu", _
"Mandriva", _
"Red Hat", _
"Fedora", _
"Gentoo" _
}
Dim fixed As New Fixed
Dim cb As New ComboBox(distros)
AddHandler cb.Changed, AddressOf Me.OnChanged
lbl = New Label("...")
fixed.Put(cb, 50, 40)
fixed.Put(lbl, 50, 140)
Me.Add(fixed)
End Sub
Private Sub OnChanged(ByVal sender As Object, _
ByVal args As EventArgs)
lbl.Text = sender.ActiveText
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim distros() As String = New String() { _This is an array of strings, that will be shown in the ComboBox widget.
"Ubuntu", _
"Mandriva", _
"Red Hat", _
"Fedora", _
"Gentoo" _
}
Dim cb As New ComboBox(distros)The ComboBox widget is created. The constructor takes the array of strings as a parameter.
Private Sub OnChanged(ByVal sender As Object, _Inside the OnChanged method, we get the selected text out of the combo box and set it to the label.
ByVal args As EventArgs)
lbl.Text = sender.ActiveText
End Sub
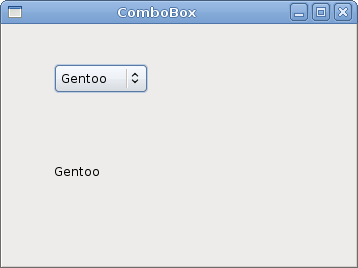
Figure: ComboBox
In this chapter, we showed some basic widgets of the GTK# programming library with the Visual Basic language.
No comments:
Post a Comment