Events in Java Gnome
In this part of the Java Gnome programming tutorial, we will talk about events.Java Gnome library is an event driven system. All GUI applications are event driven. The applications start a main loop, which continuously checks for newly generated events. If there is no event, the application waits and does nothing.
Simple event example
The next example shows, how we react to two basic events.quitbutton.java
package com.zetcode;In our code example, we react to two events. Delete event and click event. The delete event is triggered, when we close the window. By default, the window is destroyed but the application does not quit. We must explicitly quit the program.
import org.gnome.gdk.Event;
import org.gnome.gtk.Button;
import org.gnome.gtk.Fixed;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* Java Gnome tutorial
*
* This program demonstrates two
* basic events.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GButton extends Window {
public GButton() {
setTitle("Button");
initUI();
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
Fixed fixed = new Fixed();
Button quit = new Button("Quit");
quit.connect(new Button.Clicked() {
public void onClicked(Button button) {
Gtk.mainQuit();
}
});
quit.setSizeRequest(80, 35);
fixed.put(quit, 50, 50);
add(fixed);
setSizeRequest(250, 200);
}
public static void main(String[] args) {
Gtk.init(args);
new GButton();
Gtk.main();
}
}
connect(new Window.DeleteEvent() {If we click on the close button of the window, the window is destroyed. But the application is not fully destroyed. We must call the Gtk.mainQuit() to end the application. We use the connect() method to connect a callback method to a specific event type. In our case it is the DeleteEvent.
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
quit.connect(new Button.Clicked() {When we click on the button widget, the onClicked()method is triggered. We quit the application in reaction to the button click.
public void onClicked(Button button) {
Gtk.mainQuit();
}
});
Moving window
The next example shows, how we react to move events of a window. We show the current position of the upper left corner of the window in the titlebar.move.java
package com.zetcode;Resizing and moving windows end up in ConfigureEvent being created.
import org.gnome.gdk.Event;
import org.gnome.gdk.EventConfigure;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* ZetCode Java Gnome tutorial
*
* This program demonstrates the
* configure event.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GMoveWindow extends Window
implements Window.ConfigureEvent {
public GMoveWindow() {
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
connect(this);
setPosition(WindowPosition.CENTER);
setTitle("");
resize(250, 200);
showAll();
}
public boolean onConfigureEvent(Widget widget,
EventConfigure eventConfigure) {
int x = eventConfigure.getX();
int y = eventConfigure.getY();
setTitle(x + ", " + y);
return false;
}
public static void main(String[] args) {
Gtk.init(args);
new GMoveWindow();
Gtk.main();
}
}
public boolean onConfigureEvent(Widget widget,We set the x, y coordinates of the window to the titlebar of the window.
EventConfigure eventConfigure) {
int x = eventConfigure.getX();
int y = eventConfigure.getY();
setTitle(x + ", " + y);
return false;
}
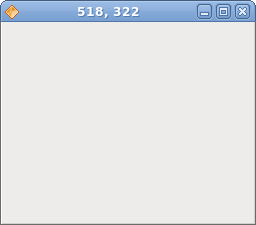
Figure: Move event
EnterNotifyEvent
EnterNotifyEventis emitted, when we enter the area of a widget with a mouse pointer.enter.java
package com.zetcode;We will change the background color of the button widget, once we hover a mouse pointer over it.
import org.gnome.gdk.Color;
import org.gnome.gdk.Event;
import org.gnome.gdk.EventCrossing;
import org.gnome.gtk.Button;
import org.gnome.gtk.Fixed;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.StateType;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* ZetCode Java Gnome tutorial
*
* This program demonstrates the
* EnterNotifyEvent.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GEnterNotifyEvent extends Window {
private Color lightGray = new Color(55000, 55000, 55000);
public GEnterNotifyEvent() {
setTitle("EnterNotifyEvent");
initUI();
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setDefaultSize(250, 150);
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
Button button = new Button("Button");
button.setSizeRequest(80, 30);
button.connect(new Button.EnterNotifyEvent() {
public boolean onEnterNotifyEvent(Widget widget,
EventCrossing eventCrossing) {
widget.modifyBackground(StateType.PRELIGHT, lightGray);
return false;
}
});
Fixed fix = new Fixed();
fix.put(button, 20, 20);
add(fix);
}
public static void main(String[] args) {
Gtk.init(args);
new GEnterNotifyEvent();
Gtk.main();
}
}
button.connect(new Button.EnterNotifyEvent() {Here we react to the EnterNotifyEvent.
public boolean onEnterNotifyEvent(Widget widget,
EventCrossing eventCrossing) {
widget.modifyBackground(StateType.PRELIGHT, lightGray);
return false;
}
});
widget.modifyBackground(StateType.PRELIGHT, lightGray);We modify the color of the button's background.
This chapter was about events in Java Gnome.
No comments:
Post a Comment