First steps in Java Gnome
In this part of the Java Gnome programming tutorial, we will do our first steps in programming. We will create simple programs.Simple example
The first code example is a simple one that shows a centered window.simple.java
package com.zetcode;The code example shows a small window in the center of the screen.
import org.gnome.gdk.Event;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* ZetCode Java Gnome tutorial
*
* This program is a simple Java Gnome
* application.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GSimple extends Window {
public GSimple() {
setTitle("Simple");
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setDefaultSize(250, 150);
setPosition(WindowPosition.CENTER);
show();
}
public static void main(String[] args) {
Gtk.init(args);
new GSimple();
Gtk.main();
}
}
import org.gnome.gdk.Event;We import all necessary objects.
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
public class GSimple extends Window {Our application is based on the Window class. Window is a toplevel widget that is used as a container for other widgets.
setTitle("Simple");Here we set the title for the window.
connect(new Window.DeleteEvent() {This code cleanly exits the Java Gnome application, if we close the window.
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setDefaultSize(250, 150);This line sets a default size for the window.
setPosition(WindowPosition.CENTER);This line centers the window on the screen.
show();Now we show the window. The window is not visible, until we call the show() method.
public static void main(String[] args) {The main() method is the entry point to the application. It initiates and runs the program.
Gtk.init(args);
new GSimple();
Gtk.main();
}
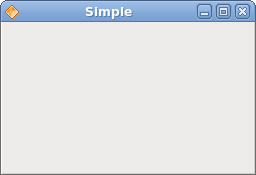
Figure: Simple
Icon
In the next example, we show the application icon. Most window managers display the icon in the left corner of the titlebar and also on the taskbar.icon.java
package com.zetcode;The code example shows the application icon.
import java.io.FileNotFoundException;
import org.gnome.gdk.Event;
import org.gnome.gdk.Pixbuf;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* ZetCode Java Gnome tutorial
*
* This program shows a small icon
* in the top left corner of the window.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GIcon extends Window implements Window.DeleteEvent {
Pixbuf icon;
public GIcon() {
setTitle("Icon");
initUI();
connect(this);
setDefaultSize(250, 150);
setPosition(WindowPosition.CENTER);
show();
}
public void initUI() {
try {
icon = new Pixbuf("web.png");
} catch (FileNotFoundException e) {
e.printStackTrace();
}
setIcon(icon);
}
public boolean onDeleteEvent(Widget widget, Event event) {
Gtk.mainQuit();
return false;
}
public static void main(String[] args) {
Gtk.init(args);
new GIcon();
Gtk.main();
}
}
initUI();We delegate the setup of the user interface to the initUI() method.
try {We load a pixbuf from the file on the disk.
icon = new Pixbuf("web.png");
} catch (FileNotFoundException e) {
e.printStackTrace();
}
setIcon(icon);The setIcon() method sets an icon for the window.
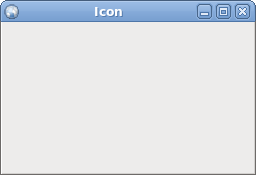
Figure: Icon
Buttons
In the next example, we will further enhance our programming skills with the Java Gnome library.buttons.java
package com.zetcode;We show four different buttons on the window. We will see a difference between container widgets and child widgets and will change some properties of child widgets.
import org.gnome.gdk.Event;
import org.gnome.gtk.Button;
import org.gnome.gtk.Fixed;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Stock;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* ZetCode Java Gnome tutorial
*
* This program shows four buttons
* with different characteristics.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GButtons extends Window {
public GButtons() {
setTitle("Buttons");
initUI();
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setDefaultSize(250, 200);
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
Fixed fix = new Fixed();
Button btn1 = new Button("Button");
btn1.setSensitive(false);
Button btn2 = new Button("Button");
Button btn3 = new Button(Stock.CLOSE);
Button btn4 = new Button("Button");
btn4.setSizeRequest(80, 40);
fix.put(btn1, 20, 30);
fix.put(btn2, 100, 30);
fix.put(btn3, 20, 80);
fix.put(btn4, 100, 80);
add(fix);
}
public static void main(String[] args) {
Gtk.init(args);
new GButtons();
Gtk.main();
}
}
Fixed fix = new Fixed();Fixed widget is a non visible container widget. It's purpose is to contain other child widgets.
Button btn1 = new Button("Button");A Button is a child widget. Child widgets are placed inside containers.
btn1.setSensitive(false);We make this button insensitive. This means, we cannot click on it. Graphically the widget is grayed out.
Button btn3 = new Button(Stock.CLOSE);The third button shows an image inside it's area. The Java Gnome library has a built-in stock of images, that we can use.
btn4.setSizeRequest(80, 40);Here we change the size of the button.
fix.put(btn1, 20, 30);Here we place button widgets inside fixed container widget.
fix.put(btn2, 100, 30);
...
add(fix);We set the Fixed container to be the main container for our Window widget.
showAll();We can either call showAll() method, which shows all widgets at once, or we call show() method on each of the widgets. Including containers.
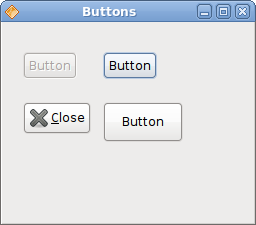
Figure: Buttons
In this chapter, we created first programs in Java Gnome programming library.
No comments:
Post a Comment