Dialogs
In this part of the Visual Basic GTK# programming tutorial, we will introduce dialogs.Dialog windows or dialogs are an indispensable part of most modern GUI applications. A dialog is defined as a conversation between two or more persons. In a computer application a dialog is a window which is used to "talk" to the application. A dialog is used to input data, modify data, change the application settings etc. Dialogs are important means of communication between a user and a computer program.
Message boxes
Message dialogs are convenient dialogs that provide messages to the user of the application. The message consists of textual and image data.' ZetCode Mono Visual Basic GTK# tutorialIn our example, we will show four kinds of message dialogs. Information, Warning, Question and Error message dialogs.
'
' This program shows message dialogs.
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Public Sub New
MyBase.New("Message dialogs")
Me.InitUI
Me.SetDefaultSize(250, 100)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim table As New Table(2, 2, True)
Dim info As New Button("Information")
Dim warn As New Button("Warning")
Dim ques As New Button("Question")
Dim erro As New Button("Error")
AddHandler info.Clicked, AddressOf Me.OnInfo
AddHandler warn.Clicked, AddressOf Me.OnWarning
AddHandler ques.Clicked, AddressOf Me.OnQuestion
AddHandler erro.Clicked, AddressOf Me.OnError
table.Attach(info, 0, 1, 0, 1)
table.Attach(warn, 1, 2, 0, 1)
table.Attach(ques, 0, 1, 1, 2)
table.Attach(erro, 1, 2, 1, 2)
Me.Add(table)
End Sub
Private Sub OnInfo(ByVal sender As Object, ByVal args As EventArgs)
Dim md As MessageDialog = New MessageDialog(Me, _
DialogFlags.DestroyWithParent, MessageType.Info, _
ButtonsType.Close, "Download completed")
md.Run
md.Destroy
End Sub
Private Sub OnWarning(ByVal sender As Object, ByVal args As EventArgs)
Dim md As MessageDialog = New MessageDialog(Me, _
DialogFlags.DestroyWithParent, MessageType.Warning, _
ButtonsType.Close, "Unallowed operation")
md.Run
md.Destroy
End Sub
Private Sub OnQuestion(ByVal sender As Object, ByVal args As EventArgs)
Dim md As MessageDialog = New MessageDialog(Me, _
DialogFlags.DestroyWithParent, MessageType.Question, _
ButtonsType.Close, "Are you sure to quit?")
md.Run
md.Destroy
End Sub
Private Sub OnError(ByVal sender As Object, ByVal args As EventArgs)
Dim md As MessageDialog = New MessageDialog(Me, _
DialogFlags.DestroyWithParent, MessageType.Error, _
ButtonsType.Close, "Error loading file")
md.Run
md.Destroy
End Sub
Private Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim info As New Button("Information")We have four buttons. Each of these buttons will show a different kind of message dialog.
Dim warn As New Button("Warning")
Dim ques As New Button("Question")
Dim erro As New Button("Error")
Private Sub OnInfo(ByVal sender As Object, ByVal args As EventArgs)If we click on the info button, the Information dialog is displayed. The MessageType.Info specifies the type of the dialog. The ButtonsType.Close specifies the button to be displayed in the dialog. The last parameter is the message dislayed. The dialog is displayed with the Run method. The programmer must also call either the Destroy or the Hide method.
Dim md As MessageDialog = New MessageDialog(Me, _
DialogFlags.DestroyWithParent, MessageType.Info, _
ButtonsType.Close, "Download completed")
md.Run
md.Destroy
End Sub
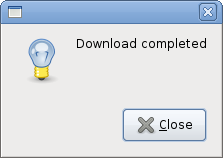
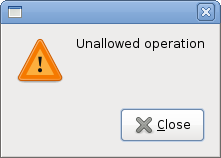
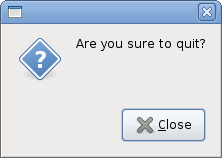
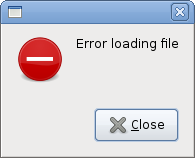
AboutDialog
The AboutDialog displays information about the application. AboutDialogcan display a logo, the name of the application, version, copyright, website or licence information. It is also possible to give credits to the authors, documenters, translators and artists.' ZetCode Mono Visual Basic GTK# tutorialThe code example uses a AboutDialog with some of its features.
'
' This program shows the about
' dialog
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Public Sub New
MyBase.New("About dialog")
Me.InitUI
Me.SetDefaultSize(350, 300)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim button As New Button("About")
AddHandler button.Clicked, AddressOf Me.ShowDialog
Dim fixed As New Fixed
fixed.Put(button, 20, 20)
Me.Add(fixed)
End Sub
Private Sub ShowDialog(ByVal sender As Object, _
ByVal args As EventArgs)
Dim about As New AboutDialog
about.ProgramName = "Battery"
about.Version = "0.1"
about.Copyright = "(c) Jan Bodnar"
about.Comments = "Battery is a simple tool for battery checking"
about.Website = "http://www.zetcode.com"
about.Logo = New Gdk.Pixbuf("battery.png")
about.Run
about.Destroy
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim about As New AboutDialogWe create an AboutDialog.
Dim about As New AboutDialogBy setting the properties of the dialog, we specify the name, version and the copyright.
about.ProgramName = "Battery"
about.Version = "0.1"
about.Copyright = "(c) Jan Bodnar"
about.Logo = New Gdk.Pixbuf("battery.png")This line creates a logo.
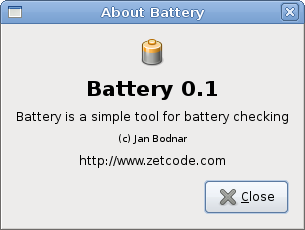
Figure: AboutDialog
FontSelectionDialog
The FontSelectionDialog is a dialog for selecting fonts. It is typically used in applications, that do some text editing or formatting.' ZetCode Mono Visual Basic GTK# tutorialIn the code example, we have a button and a label. We show the FontSelectionDialog by clicking on the button.
'
' This program shows the FontSelectionDialog
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Dim label As Label
Public Sub New
MyBase.New("Font dialog")
Me.InitUI
Me.SetDefaultSize(350, 300)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
label = New Label("The only victory over love is flight.")
Dim button As New Button("Select font")
AddHandler button.Clicked, AddressOf Me.ShowDialog
Dim fixed As New Fixed
fixed.Put(button, 100, 30)
fixed.Put(label, 30, 90)
Me.Add(fixed)
End Sub
Private Sub ShowDialog(ByVal sender As Object, _
ByVal args As EventArgs)
Dim fdia As New FontSelectionDialog("Select font name")
AddHandler fdia.Response, AddressOf Me.SelectFont
fdia.Run
fdia.Destroy
End Sub
Private Sub SelectFont(ByVal sender As Object, _
ByVal args As ResponseArgs)
If args.ResponseId = ResponseType.Ok
Dim fontdesc As Pango.FontDescription = _
Pango.FontDescription.FromString(sender.FontName)
label.ModifyFont(fontdesc)
End If
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim fdia As New FontSelectionDialog("Select font name")We create the FontSelectionDialog.
If args.ResponseId = ResponseType.OkIf we click on the OK button, the font of the label widget changes to the one, that we selected in the dialog.
Dim fontdesc As Pango.FontDescription = _
Pango.FontDescription.FromString(sender.FontName)
label.ModifyFont(fontdesc)
End If
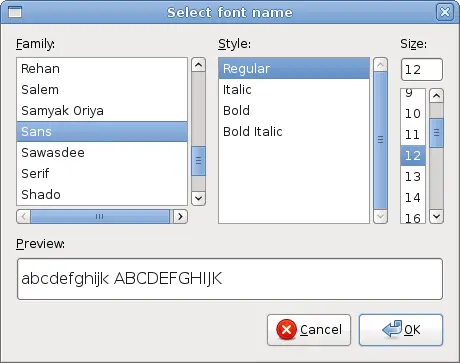
Figure: FontSelectionDialog
ColorSelectionDialog
ColorSelectionDialog is a dialog for selecting a color.' ZetCode Mono Visual Basic GTK# tutorialThe example is very similar to the previous one. This time we change the color of the label.
'
' This program shows the ColorSelectionDialog
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Dim label As Label
Public Sub New
MyBase.New("Color dialog")
Me.InitUI
Me.SetDefaultSize(350, 300)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
label = New Label("The only victory over love is flight.")
Dim button As New Button("Select color")
AddHandler button.Clicked, AddressOf Me.ShowDialog
Dim fixed As New Fixed
fixed.Put(button, 100, 30)
fixed.Put(label, 30, 90)
Me.Add(fixed)
End Sub
Private Sub ShowDialog(ByVal sender As Object, _
ByVal args As EventArgs)
Dim cdia As New ColorSelectionDialog("Select color")
AddHandler cdia.Response, AddressOf Me.SelectColor
cdia.Run
cdia.Destroy
End Sub
Private Sub SelectColor(ByVal sender As Object, _
ByVal args As ResponseArgs)
If args.ResponseId = ResponseType.Ok
label.ModifyFg(StateType.Normal, _
sender.ColorSelection.CurrentColor)
End If
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Dim cdia As New ColorSelectionDialog("Select color")We create the ColorSelectionDialog.
If args.ResponseId = ResponseType.OkIf the user pressed OK, we get the color value and modify the label's color.
label.ModifyFg(StateType.Normal, _
sender.ColorSelection.CurrentColor)
End If
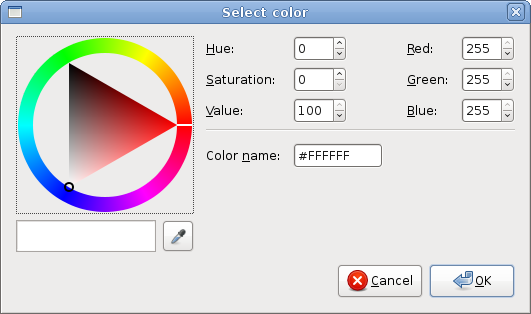
Figure: ColorSelectionDialog
In this part of the Visual Basic GTK# tutorial, we presented dialogs.
No comments:
Post a Comment