Pango
In this part of the Java Gnome programming tutorial, we will explore the Pango library.Pango is a free and open source computing library for rendering internationalized texts in high quality. Different font backends can be used, allowing cross-platform support. (wikipedia)
Pango provides advanced font and text handling that is used for Gdk and Gtk.
Quotes
In our first example, we show, how to change font for the Label widget.quotes.java
package com.zetcode;In the above code example, we have a label widget with three quotations. We change it's font to Purisa 9.
import org.gnome.gdk.Event;
import org.gnome.gtk.Fixed;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Label;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
import org.gnome.pango.FontDescription;
/**
* ZetCode Java Gnome tutorial
*
* This program uses the pango library to
* display text.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GQuotes extends Window {
public GQuotes() {
setTitle("Quotes");
initUI();
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
String text = "Excess of joy is harder to bear than any amount\n" +
"of sorrow. The more one judges, the less one loves.\n" +
"There is no such thing as a great talent without great will power.";
Label label = new Label(text);
FontDescription fontdesc = new FontDescription("Purisa 9");
label.modifyFont(fontdesc);
Fixed fix = new Fixed();
fix.put(label, 5, 5);
add(fix);
}
public static void main(String[] args) {
Gtk.init(args);
new GQuotes();
Gtk.main();
}
}
String text = "Excess of joy is harder to bear than any amount\n" +This is the text to show in the label.
"of sorrow. The more one judges, the less one loves.\n" +
"There is no such thing as a great talent without great will power.";
FontDescription fontdesc = new FontDescription("Purisa 9");The FontDescription is used to specify the characteristics of a font to load.
label.modifyFont(fontdesc);We change the font of the label widget to Purisa 9.
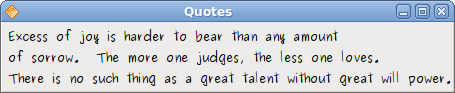
Figure: Quotations
Pango markup
In the following example, we will use Pango markup language to modify font in the ExposeEvent event.markup.java
package com.zetcode;Drawing is done with Cairo library. We get a Layout by passing the Cairo drawing Context.
import org.freedesktop.cairo.Context;
import org.gnome.gdk.Event;
import org.gnome.gdk.EventExpose;
import org.gnome.gtk.DrawingArea;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.pango.FontDescription;
import org.gnome.pango.Layout;
/**
* ZetCode Java Gnome tutorial
*
* This program uses Pango markup language
* to modify the text.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GPangoMarkup extends Window implements Widget.ExposeEvent {
String quote = "<span foreground='blue' size='19000'>The only " +
"victory over love is flight</span>";
public GPangoMarkup() {
setTitle("Pango Markup");
initUI();
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
move(150, 150);
showAll();
}
public void initUI() {
DrawingArea darea = new DrawingArea();
darea.connect(this);
darea.queueDraw();
add(darea);
setBorderWidth(5);
}
public boolean onExposeEvent(Widget widget, EventExpose eventExpose) {
final Context cr;
final Layout layout;
final FontDescription desc;
cr = new Context(widget.getWindow());
layout = new Layout(cr);
desc = new FontDescription("Sans 12");
layout.setFontDescription(desc);
layout.setMarkup(quote);
widget.setSizeRequest(layout.getPixelWidth(), layout.getPixelHeight());
cr.showLayout(layout);
return false;
}
public static void main(String[] args) {
Gtk.init(args);
new GPangoMarkup();
Gtk.main();
}
}
cr = new Context(widget.getWindow());This code line creates a Context object.
layout = new Layout(cr);A Layout represents a paragraph of text, together with its attributes.
layout.setMarkup(quote);This line sets the markup for the layout.
widget.setSizeRequest(layout.getPixelWidth(), layout.getPixelHeight());This is to show all the text in the window.
cr.showLayout(layout);Finally, the text is drawn.

Figure: Pango markup
Unicode
Pango is used to work with internationalized text.unicode.java
package com.zetcode;We show some text in azbuka.
import org.gnome.gdk.Event;
import org.gnome.gtk.Fixed;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Label;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
import org.gnome.pango.FontDescription;
/**
* ZetCode Java Gnome tutorial
*
* This program shows text in
* azbuka.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GUnicode extends Window {
public GUnicode() {
setTitle("Unicode");
initUI();
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
String text =
"Фёдор Михайлович Достоевский родился 30 октября (11 ноября)\n" +
"1821 года в Москве.Был вторым из 7 детей. Отец, Михаил Андреевич,\n" +
"работал в госпитале для бедных. Мать, Мария Фёдоровна\n" +
"(в девичестве Нечаева), происходила из купеческого рода.";
Label label = new Label(text);
FontDescription fontdesc = new FontDescription("Purisa 9");
label.modifyFont(fontdesc);
Fixed fix = new Fixed();
fix.put(label, 5, 5);
add(fix);
setBorderWidth(5);
}
public static void main(String[] args) {
Gtk.init(args);
new GUnicode();
Gtk.main();
}
}
String text =We can directly use unicode text. However, text is never put inside the source code. In real worl applications, we put text in external files. In Java these are often property files.
"Фёдор Михайлович Достоевский родился 30 октября (11 ноября)\n" ...
Label label = new Label(text);We normally use it in the label widget.
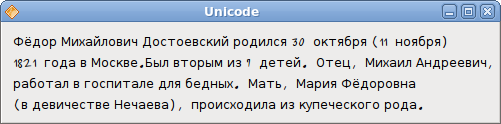
Figure: Unicode
In this chapter of the Java Gnome programming tutorial, we worked with the Pango library.
No comments:
Post a Comment