Dialogs in Java Gnome
In this part of the Java Gnome programming tutorial, we will introduce dialogs.Dialog windows or dialogs are an indispensable part of most modern GUI applications. A dialog is defined as a conversation between two or more persons. In a computer application a dialog is a window which is used to "talk" to the application. A dialog is used to input data, modify data, change the application settings etc. Dialogs are important means of communication between a user and a computer program.
Message dialogs
Message dialogs are convenient dialogs that provide messages to the user of the application. The message consists of textual and image data.messages.java
package com.zetcode;In our example, we will show four kinds of message dialogs. Information, Warning, Question and Error message dialogs.
import org.gnome.gdk.Event;
import org.gnome.gtk.Button;
import org.gnome.gtk.ButtonsType;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.MessageDialog;
import org.gnome.gtk.MessageType;
import org.gnome.gtk.Table;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
/**
* Java Gnome tutorial
*
* This program shows four
* message dialogs.
*
* @author jan bodnar
* website zetcode.com
* last modified March 2009
*/
public class GMessages extends Window {
Window parent;
public GMessages() {
setTitle("GMessages");
initUI();
parent = this;
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setDefaultSize(250, 100);
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
Table table = new Table(2, 2, true);
Button info = new Button("Information");
Button warn = new Button("Warning");
Button ques = new Button("Question");
Button erro = new Button("Error");
info.connect(new Button.Clicked() {
public void onClicked(Button button) {
MessageDialog md = new MessageDialog(null, true,
MessageType.INFO,
ButtonsType.CLOSE, "Download completed");
md.setPosition(WindowPosition.CENTER);
md.run();
md.hide();
}
});
warn.connect(new Button.Clicked() {
public void onClicked(Button button) {
MessageDialog md = new MessageDialog(parent, true,
MessageType.WARNING,
ButtonsType.CLOSE, "Unallowed operation");
md.setPosition(WindowPosition.CENTER);
md.run();
md.hide();
}
});
ques.connect(new Button.Clicked() {
public void onClicked(Button button) {
MessageDialog md = new MessageDialog(null, true,
MessageType.QUESTION,
ButtonsType.CLOSE, "Are you sure to quit?");
md.setPosition(WindowPosition.CENTER);
md.run();
md.hide();
}
});
erro.connect(new Button.Clicked() {
public void onClicked(Button button) {
MessageDialog md = new MessageDialog (null, true,
MessageType.ERROR,
ButtonsType.CLOSE, "Error loading file");
md.setPosition(WindowPosition.CENTER);
md.run();
md.hide();
}
});
table.attach(info, 0, 1, 0, 1);
table.attach(warn, 1, 2, 0, 1);
table.attach(ques, 0, 1, 1, 2);
table.attach(erro, 1, 2, 1, 2);
add(table);
}
public static void main(String[] args) {
Gtk.init(args);
new GMessages();
Gtk.main();
}
}
Button info = new Button("Information");We have four buttons. Each of these buttons will show a different kind of message dialog.
Button warn = new Button("Warning");
Button ques = new Button("Question");
Button erro = new Button("Error");
info.connect(new Button.Clicked() {If we click on the info button, the Information dialog is displayed. The MessageType.INFO specifies the type of the dialog. The ButtonsType.CLOSE specifies the button to be displayed in the dialog. The last parameter is the message dislayed. The dialog is displayed with the run() method. The hide() method hides the dialog.
public void onClicked(Button button) {
MessageDialog md = new MessageDialog(null, true,
MessageType.INFO,
ButtonsType.CLOSE, "Download completed");
md.setPosition(WindowPosition.CENTER);
md.run();
md.hide();
}
});
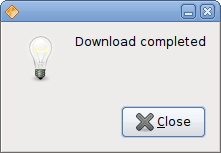
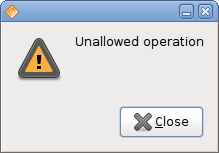
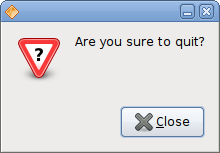
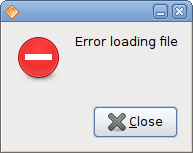
AboutDialog
The AboutDialog displays information about the application. AboutDialog can display a logo, the name of the application, version, copyright or licence information. It is also possible to give credits to the authors or translators.aboutdialog.java
package com.zetcode;The code example uses a AboutDialog with some of it's features.
import java.io.FileNotFoundException;
import org.gnome.gdk.Event;
import org.gnome.gdk.Pixbuf;
import org.gnome.gtk.AboutDialog;
import org.gnome.gtk.Button;
import org.gnome.gtk.Fixed;
import org.gnome.gtk.Gtk;
import org.gnome.gtk.Widget;
import org.gnome.gtk.Window;
import org.gnome.gtk.WindowPosition;
public class GAboutDialog extends Window implements Button.Clicked {
Pixbuf logo;
public GAboutDialog() {
setTitle("AboutDialog");
initUI();
connect(new Window.DeleteEvent() {
public boolean onDeleteEvent(Widget source, Event event) {
Gtk.mainQuit();
return false;
}
});
setDefaultSize(250, 200);
setPosition(WindowPosition.CENTER);
showAll();
}
public void initUI() {
try {
logo = new Pixbuf("battery.png");
} catch (FileNotFoundException e) {
e.printStackTrace();
}
Button button = new Button("About");
button.connect(this);
Fixed fix = new Fixed();
fix.put(button, 20, 20);
add(fix);
}
public void onClicked(Button button) {
AboutDialog about = new AboutDialog();
about.setProgramName("Battery");
about.setVersion("0.1");
about.setCopyright("(c) Jan Bodnar");
about.setComments("Battery is a simple tool for battery checking");
about.setLogo(logo);
about.setPosition(WindowPosition.CENTER);
about.run();
about.hide();
}
public static void main(String[] args) {
Gtk.init(args);
new GAboutDialog();
Gtk.main();
}
}
AboutDialog about = new AboutDialog();We create an AboutDialog.
about.setProgramName("Battery");We set the name, version and the copyright.
about.setVersion("0.1");
about.setCopyright("(c) Jan Bodnar");
about.setLogo(logo);This line creates a logo.
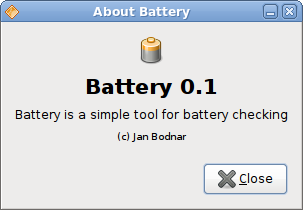
Figure: AboutDialog
In this part of the Java Gnome tutorial, we have covered Dialogs.
No comments:
Post a Comment