Introduction to Visual Basic GTK#
In this part of the Visual Basic GTK# programming tutorial, we will introduce the GTK# library and create our first programs using the Visual Basic programming language.The purpose of this tutorial is to get you started with the GTK# and Visual Basic. Images used in this tutorial can be downloaded here. I used some icons from the tango icons pack of the Gnome project.
About
GTK# is a library that provides binding of the GTK+ to the Mono .NET languages like C# or Visual Basic. GTK+ is one of the leading toolkits for creating graphical user interfaces. Both GTK# and Visual Basic are parts of the Mono development platform.vbnc -r:/usr/lib/mono/gtk-sharp-2.0/gtk-sharp.dll quitbutton.vbThe above command shows, how to compile the quitbutton example. The -r parameter of the mono VB compiler loads the GTK# assembly. It is a dynamic library. The commnad shows a path to the dll library on a Ubuntu system.
Simple example
In the first example, we create a simple window. The window is centered on the screen.' ZetCode Mono Visual Basic GTK# tutorialThis example shows a 250x150 px window in the centre of the screen.
'
' This program centers a window on
' the screen
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Public Sub New
MyBase.New("Center")
Me.SetDefaultSize(250, 150)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDeleteEvent
Me.Show
End Sub
Sub OnDeleteEvent(ByVal sender as Object, _
ByVal args as DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Imports GtkThe Imports keyword imports necessery types that we will use in the application.
Public Class GtkVBAppThe example inherits from a Window. The Window is a toplevel container.
Inherits Window
MyBase.New("Center")Here we call the parent's constructor.
Me.SetDefaultSize(250, 150)We set a default size for the application window.
Me.SetPosition(WindowPosition.Center)This line centers the window on the screen.
AddHandler Me.DeleteEvent, AddressOf Me.OnDeleteEventWe plug a handler to the DeleteEvent.
Me.ShowWhen everything is ready, we show the window on the screen.
Sub OnDeleteEvent(ByVal sender as Object, _The event is triggered, when we click on the close button in the titlebar. Or press Alt + F4. The method quits the application for good.
ByVal args as DeleteEventArgs)
Application.Quit
End Sub
Application.InitThese three lines set up the application.
Dim app As New GtkVBApp
Application.Run
Creating a Tooltip
The second example will show a tooltip. A tooltip is a small rectangular window, which gives a brief information about an object. It is usually a GUI component. It is part of the help system of the application.' ZetCode Mono Visual Basic GTK# tutorialThe example creates a window. If we hover a mouse pointer over the area of the window, a tooltip pops up.
'
' This program shows a tooltip
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Public Sub New
MyBase.New("Tooltip")
Me.SetDefaultSize(250, 150)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDeleteEvent
Me.TooltipText = "This is a Window"
Me.Show
End Sub
Sub OnDeleteEvent(ByVal sender as Object, _
ByVal args as DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Me.TooltipText = "This is a Window"We set a tooltip through the TooltipText property.
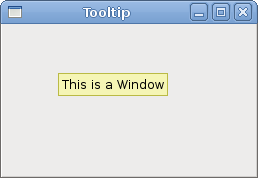
Figure: Tooltip
Quit button
In the last example of this section, we will create a quit button. When we press this button, the application terminates.' ZetCode Mono Visual Basic GTK# tutorialWe use a Button widget. This is a very common widget. It shows a text label, image or both.
'
' This program creates a quit
' button. When we press the button,
' the application terminates.
'
' author jan bodnar
' last modified May 2009
' website www.zetcode.com
Imports Gtk
Public Class GtkVBApp
Inherits Window
Public Sub New
MyBase.New("Quit button")
Me.InitUI
Me.SetDefaultSize(250, 150)
Me.SetPosition(WindowPosition.Center)
AddHandler Me.DeleteEvent, AddressOf Me.OnDelete
Me.ShowAll
End Sub
Private Sub InitUI
Dim quitButton As New Button("Quit")
quitButton.SetSizeRequest(80, 30)
AddHandler quitButton.Clicked, AddressOf Me.OnQuit
Dim fix As New Fixed
fix.Put(quitButton, 50, 50)
Me.Add(fix)
End Sub
Sub OnQuit(ByVal sender As Object, _
ByVal args As EventArgs)
Application.Quit
End Sub
Sub OnDelete(ByVal sender As Object, _
ByVal args As DeleteEventArgs)
Application.Quit
End Sub
Public Shared Sub Main
Application.Init
Dim app As New GtkVBApp
Application.Run
End Sub
End Class
Me.InitUIWe delegate the creation of the user interface to the InitUI method.
Me.ShowAllWe have two options. Either to call Show on all widgets, or to call ShowAll, which shows the container and all its children.
Dim quitButton As New Button("Quit")Here we create a button widget.
quitButton.SetSizeRequest(80, 30)We set a size for a button.
AddHandler quitButton.Clicked, AddressOf Me.OnQuitWe plug the OnQuit method to the button Clicked event.
Dim fix As New FixedWe put the quit button into the fixed container at x=50, y=50.
fix.Put(quitButton, 50, 50)
Sub OnQuit(ByVal sender As Object, _Inside the the OnQuit method, we terminate the application.
ByVal args As EventArgs)
Application.Quit
End Sub
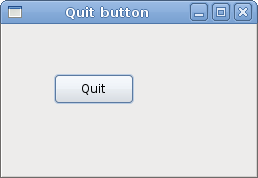
Figure: Quit button
This section was an introduction to the GTK# library with the Visual Basic language.
No comments:
Post a Comment