Introduction to Ruby GTK
In this part of the Ruby GTK programming tutorial, we will introduce the GTK library and create our first programs using the Ruby programming language.The purpose of this tutorial is to get you started with the GTK and Ruby. Images for the Nibbles game can be downloaded here.
About
GTK is one of the leading toolkits for creating graphical user interfaces. Ruby is a popular scripting language.Simple example
In the first example, we create a simple window. The window is centered on the screen.#!/usr/bin/rubyThis example shows a 250x200 px window in the centre of the screen.
# ZetCode Ruby GTK tutorial
#
# This program centers a window on
# the screen
#
# author: jan bodnar
# website: www.zetcode.com
# last modified: April 2009
require 'gtk2'
class RubyApp < Gtk::Window
def initialize
super
set_title "Center"
signal_connect "destroy" do
Gtk.main_quit
end
set_default_size 250, 200
set_window_position Gtk::Window::POS_CENTER
show
end
end
Gtk.init
window = RubyApp.new
Gtk.main
require 'gtk2'The
require
keyword imports necessery types that we will use in the application. class RubyApp < Gtk::WindowThe example inherits from a
Window
. The Window is a toplevel container. set_title "Center"We set a title for the window.
signal_connect "destroy" doThe
Gtk.main_quit
end
destroy
event is triggered, when we click on the close button in the titlebar. Or press Alt + F4. The method main_quit
quits the application for good. set_default_size 250, 200We set a default size for the application window.
set_window_position Gtk::Window::POS_CENTERThis line centers the window on the screen.
showWhen everything is ready, we show the window on the screen.
Gtk.initThese three lines set up the application.
window = RubyApp.new
Gtk.main
Creating a Tooltip
The second example will show a tooltip. A tooltip is a small rectangular window, which gives a brief information about an object. It is usually a GUI component. It is part of the help system of the application.#!/usr/bin/rubyThe example creates a window. If we hover a mouse pointer over the area of the window, a tooltip pops up.
# ZetCode Ruby GTK tutorial
#
# This code shows a tooltip on
# a window and a button
#
# author: jan bodnar
# website: www.zetcode.com
# last modified: June 2009
require 'gtk2'
class RubyApp < Gtk::Window
def initialize
super
set_title "Tooltips"
signal_connect "destroy" do
Gtk.main_quit
end
fixed = Gtk::Fixed.new
add fixed
button = Gtk::Button.new "Button"
button.set_size_request 80, 35
button.set_tooltip_text "Button widget"
fixed.put button, 50, 50
set_tooltip_text "Window widget"
set_default_size 250, 200
set_window_position Gtk::Window::POS_CENTER
show_all
end
end
Gtk.init
window = RubyApp.new
Gtk.main
button.set_tooltip_text "Button widget"We set a tooltip with the
set_tooltip_text
method. 
Figure: Tooltip
Quit button
In the last example of this section, we will create a quit button. When we press this button, the application terminates.#!/usr/bin/rubyWe use a
# ZetCode Ruby GTK tutorial
#
# This program creates a quit
# button. When we press the button,
# the application terminates.
#
# author: jan bodnar
# website: www.zetcode.com
# last modified: June 2009
require 'gtk2'
class RubyApp < Gtk::Window
def initialize
super
set_title "Quit button"
signal_connect "destroy" do
Gtk.main_quit
end
init_ui
show_all
end
def init_ui
fixed = Gtk::Fixed.new
add fixed
button = Gtk::Button.new "Quit"
button.set_size_request 80, 35
button.signal_connect "clicked" do
Gtk.main_quit
end
fixed.put button, 50, 50
set_default_size 250, 200
set_window_position Gtk::Window::POS_CENTER
end
end
Gtk.init
window = RubyApp.new
Gtk.main
Button
widget. This is a very common widget. It shows a text label, image or both. init_uiWe delegate the creation of the user interface to the
init_ui
method. show_allWe have two options. Either to call
show
on all widgets, or to call show_all
, which shows the container and all its children. button = Gtk::Button.new "Quit"Here we create a button widget.
button.set_size_request 80, 35We set a size for a button.
button.signal_connect "clicked" doWe plug the
Gtk.main_quit
end
main_quit
method to the button clicked
event. fixed.put button, 50, 50We put the quit button into the fixed container at x=50, y=50.
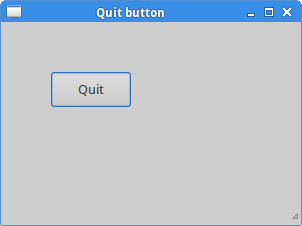
Figure: Quit button
This section was an introduction to the GTK library with the Ruby language.
No comments:
Post a Comment