Menus And toolbars
In this part of the JRuby Swing programming tutorial, we will work with menus and toolbar.A menubar is one of the most visible parts of the GUI application. It is a group of commands located in various menus. While in console applications you had to remember all those arcane commands, here we have most of the commands grouped into logical parts. There are accepted standards that further reduce the amount of time spending to learn a new application. Menus group commands that we can use in an application. Toolbars provide a quick access to the most frequently used commands.
Simple menu
The first example will show a simple menu.#!/usr/local/bin/jrubyOur example will show a menu with one item. By selecting the exit menu item we close the application.
# ZetCode JRuby Swing tutorial
#
# This program creates a simple
# menu.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import java.awt.event.KeyEvent
import javax.swing.JButton
import javax.swing.JFrame
import javax.swing.JMenuBar
import javax.swing.JMenuItem
import javax.swing.JMenu
import javax.swing.ImageIcon
import java.lang.System
class Example < JFrame
def initialize
super "Simple menu"
self.initUI
end
def initUI
menubar = JMenuBar.new
icon = ImageIcon.new "exit.png"
fileMenu = JMenu.new "File"
fileMenu.setMnemonic KeyEvent::VK_F
itemExit = JMenuItem.new "Exit", icon
itemExit.addActionListener do |e|
System.exit 0
end
itemExit.setMnemonic KeyEvent::VK_C
itemExit.setToolTipText "Exit application"
fileMenu.add itemExit
menubar.add fileMenu
self.setJMenuBar menubar
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setSize 250, 200
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
menubar = JMenuBar.newHere we create a menubar.
icon = ImageIcon.new "exit.png"We will display an icon in the menu item.
fileMenu = JMenu.new "File"We create a menu object. A menu is a popup window containing
fileMenu.setMnemonic KeyEvent::VK_F
JMenuItem
s. Menus are located on the menubar. The menus can be accessed via the keyboard as well. To bind a menu to a particular key, we use the setMnemonic
method. In our case, the menu can be opened with the ALT + F shortcut. itemExit = JMenuItem.new "Close", iconHere we create a
itemExit.addActionListener do |e|
System.exit 0
end
JMenuItem
. A menu item is an object shown in a popup window of the selected menu. We also provide a shortcut for the menu item and a tooltip as well. fileMenu.add itemExitA menu item is added to the menu.
menubar.add fileMenuA menu is added to the menubar.
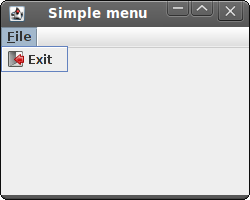
Figure: Simple menu
Submenu
A submenu is a menu plugged into another menu object. The next example demonstrates this.#!/usr/local/bin/jrubyIn the example, we have three options in a submenu of a file menu.
# ZetCode JRuby Swing tutorial
#
# This program creates a
# submenu.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import java.awt.event.KeyEvent
import java.awt.event.ActionEvent
import javax.swing.JFrame
import javax.swing.ImageIcon
import javax.swing.JMenuBar
import javax.swing.JMenu
import javax.swing.JMenuItem
import javax.swing.KeyStroke
import java.lang.System
class Example < JFrame
def initialize
super "Submenu"
self.initUI
end
def initUI
menubar = JMenuBar.new
iconNew = ImageIcon.new "new.png"
iconOpen = ImageIcon.new "open.png"
iconSave = ImageIcon.new "save.png"
iconExit = ImageIcon.new "exit.png"
fileMenu = JMenu.new "File"
fileMenu.setMnemonic KeyEvent::VK_F
imp = JMenu.new "Import"
imp.setMnemonic KeyEvent::VK_M
newsf = JMenuItem.new "Import newsfeed list..."
bookm = JMenuItem.new "Import bookmarks..."
mail = JMenuItem.new "Import mail..."
imp.add newsf
imp.add bookm
imp.add mail
fileNew = JMenuItem.new "New", iconNew
fileNew.setMnemonic KeyEvent::VK_N
fileOpen = JMenuItem.new "Open", iconOpen
fileNew.setMnemonic KeyEvent::VK_O
fileSave = JMenuItem.new "Save", iconSave
fileSave.setMnemonic KeyEvent::VK_S
fileExit = JMenuItem.new "Exit", iconExit
fileExit.addActionListener do |e|
System.exit 0
end
fileExit.setMnemonic KeyEvent::VK_C
fileExit.setToolTipText "Exit application"
fileExit.setAccelerator KeyStroke.getKeyStroke KeyEvent::VK_W,
ActionEvent::CTRL_MASK
fileMenu.add fileNew
fileMenu.add fileOpen
fileMenu.add fileSave
fileMenu.addSeparator
fileMenu.add imp
fileMenu.addSeparator
fileMenu.add fileExit
menubar.add fileMenu
self.setJMenuBar menubar
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setSize 320, 220
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
imp = JMenu.new "Import"A submenu is just like any other normal menu. It is created the same way. We simply add a menu to existing menu.
...
fileMenu.add imp
fileExit.setAccelerator KeyStroke.getKeyStroke KeyEvent::VK_W,An accelerator is a key shortcut that launches a menu item. In our case, by pressing Ctrl + W we close the application.
ActionEvent::CTRL_MASK
fileMenu.addSeparatorA separator is a horizontal line that visually separates the menu items. This way we can group items into some logical places.
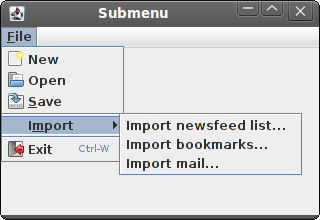
Figure: Submenu
Popup menu
In the next example, we create a popup menu.#!/usr/local/bin/jrubyIn our example, we create a popup menu with two menu items.
# ZetCode JRuby Swing tutorial
#
# This program creates a
# popup menu.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import java.awt.event.MouseAdapter
import javax.swing.JFrame
import javax.swing.JPopupMenu
import javax.swing.JMenuItem
import java.lang.System
class MouseAction < MouseAdapter
def mouseReleased e
source = e.source
menu = source.getMenu
if e.getButton == e.button
menu.show e.getComponent, e.getX, e.getY
end
end
end
class Example < JFrame
def initialize
super "Popup menu"
self.initUI
end
def initUI
@menu = JPopupMenu.new
menuItemBeep = JMenuItem.new "Beep"
menuItemBeep.addActionListener do |e|
toolkit = getToolkit
toolkit.beep
end
@menu.add menuItemBeep
menuItemExit = JMenuItem.new "Exit"
menuItemExit.addActionListener do |e|
System.exit 0
end
@menu.add menuItemExit
self.addMouseListener MouseAction.new
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setSize 250, 200
self.setLocationRelativeTo nil
self.setVisible true
end
def getMenu
@menu
end
end
Example.new
@menu = JPopupMenu.newWe create a popup menu and a menu item.
menuItemBeep = JMenuItem.new "Beep"
self.addMouseListener MouseAction.newWe add a mouse listener to the Example class. The mouse listener is a MouseAction user defined class, which inherits from a
MouseAdapter
. It is a convenience class which implements all five required methods. The methods are empty. Instead of implementing all five methods, we implement only the methods, that we need. class MouseAction < MouseAdapterIn our MouseAction class we implement the
def mouseReleased e
...
mouseReleased
method. if e.getButton == e.button:We show the popup menu window at the x, y coordinates of the mouse click.
menu.show e.getComponent, e.getX, e.getY
end
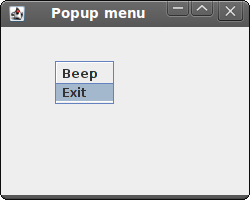
Figure: Popup menu
JToolbar
Menus group commands that we can use in an application. Toolbars provide a quick access to the most frequently used commands. In Swing, theJToolBar
class creates a toolbar in an application. #!/usr/local/bin/jrubyThe example creates a toolbar with one exit button.
# ZetCode JRuby Swing tutorial
#
# This program creates a
# toolbar.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import java.awt.BorderLayout
import javax.swing.JFrame
import javax.swing.ImageIcon
import javax.swing.JButton
import javax.swing.JMenuBar
import javax.swing.JMenu
import javax.swing.JToolBar
import java.lang.System
class Example < JFrame
def initialize
super "Toolbar"
self.initUI
end
def initUI
menubar = JMenuBar.new
fileMenu = JMenu.new "File"
menubar.add fileMenu
toolbar = JToolBar.new
iconExit = ImageIcon.new "exit2.png"
exitButton = JButton.new iconExit
exitButton.addActionListener do |e|
System.exit 0
end
toolbar.add exitButton
self.add toolbar, BorderLayout::NORTH
self.setJMenuBar menubar
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setSize 350, 250
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
toolbar = JToolBar.newA toolbar is created.
exitButton = JButton.new iconExitWe create a button and add it to the toolbar.
...
toolbar.add exitButton
self.add toolbar, BorderLayout::NORTHThe toolbar is placed into the north part of the
BorderLayout
manager. The BorderLayout
manager is the default layout manager for the JFrame
container. 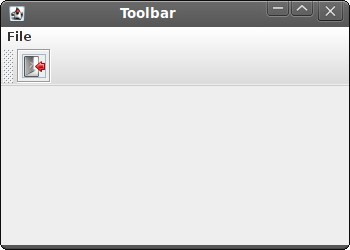
Figure: Toolbar
In this part of the JRuby Swing tutorial, we mentioned menus and toolbars.
No comments:
Post a Comment