Dialogs
In this part of the Jython Swing programming tutorial, we will work with dialogs.Dialog windows or dialogs are an indispensable part of most modern GUI applications. A dialog is defined as a conversation between two or more persons. In a computer application a dialog is a window which is used to "talk" to the application. A dialog is used to input data, modify data, change the application settings etc. Dialogs are important means of communication between a user and a computer program.
Message boxes
Message boxes are convenient dialogs that provide messages to the user of the application. The message consists of text and image data.#!/usr/local/bin/jythonWe use the
# -*- coding: utf-8 -*-
"""
ZetCode Jython Swing tutorial
In this program, we show various
message boxes.
author: Jan Bodnar
website: www.zetcode.com
last modified: November 2010
"""
from java.awt import GridLayout
from javax.swing import JButton
from javax.swing import JFrame
from javax.swing import JOptionPane
from javax.swing import JPanel
class Example(JFrame):
def __init__(self):
super(Example, self).__init__()
self.initUI()
def initUI(self):
self.panel = JPanel()
self.panel.setLayout(GridLayout(2, 2))
error = JButton("Error", actionPerformed=self.onError)
warning = JButton("Warning", actionPerformed=self.onWarning)
question = JButton("Question", actionPerformed=self.onQuestion)
inform = JButton("Information", actionPerformed=self.onInform)
self.panel.add(error)
self.panel.add(warning)
self.panel.add(question)
self.panel.add(inform)
self.add(self.panel)
self.setTitle("Message boxes")
self.setSize(300, 200)
self.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
self.setLocationRelativeTo(None)
self.setVisible(True)
def onError(self, e):
JOptionPane.showMessageDialog(self.panel, "Could not open file",
"Error", JOptionPane.ERROR_MESSAGE)
def onWarning(self, e):
JOptionPane.showMessageDialog(self.panel, "A deprecated call",
"Warning", JOptionPane.WARNING_MESSAGE)
def onQuestion(self, e):
JOptionPane.showMessageDialog(self.panel, "Are you sure to quit?",
"Question", JOptionPane.QUESTION_MESSAGE)
def onInform(self, e):
JOptionPane.showMessageDialog(self.panel, "Download completed",
"Information", JOptionPane.INFORMATION_MESSAGE)
if __name__ == '__main__':
Example()
GridLayout
manager to set up a grid of four buttons. Each of the buttons shows a different message box. def onError(self, e):In case we pressed the error button, we show the error dialog. We use the
JOptionPane.showMessageDialog(self.panel, "Could not open file",
"Error", JOptionPane.ERROR_MESSAGE)
showMessageDialog()
method to show the dialog on the screen. The first parameter of this method is the frame, in which the dialog is displayed. The second parameter is the message to be displayed. The third parameter is the title of the dialog. The final parameter is the message type. The default icon is determined by the message type. In our case, we have ERROR_MESSAGE
message type for the error dialog. 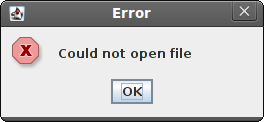
Figure: Error message dialog
JColorChooser
TheJColorChooser
is a standard dialog for selecting a color. #!/usr/local/bin/jythonIn the example, we have a white panel in the center of the window. We will change the background color of the panel by selecting a color from the color chooser dialog.
# -*- coding: utf-8 -*-
"""
ZetCode Jython Swing tutorial
In this program, we use the
JColorChooser to change the color
of a panel.
author: Jan Bodnar
website: www.zetcode.com
last modified: November 2010
"""
from java.awt import BorderLayout
from java.awt import Color
from javax.swing import BorderFactory
from javax.swing import JColorChooser
from javax.swing import JButton
from javax.swing import JToolBar
from javax.swing import JPanel
from javax.swing import JFrame
class Example(JFrame):
def __init__(self):
super(Example, self).__init__()
self.initUI()
def initUI(self):
self.panel = JPanel()
self.panel.setLayout(BorderLayout())
toolbar = JToolBar()
openb = JButton("Choose color", actionPerformed=self.onClick)
toolbar.add(openb)
self.display = JPanel()
self.display.setBackground(Color.WHITE)
self.panel.setBorder(BorderFactory.createEmptyBorder(30, 50, 30, 50))
self.panel.add(self.display)
self.add(self.panel)
self.add(toolbar, BorderLayout.NORTH)
self.setTitle("Color chooser")
self.setSize(300, 250)
self.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
self.setLocationRelativeTo(None)
self.setVisible(True)
def onClick(self, e):
clr = JColorChooser()
color = clr.showDialog(self.panel, "Choose Color", Color.white)
self.display.setBackground(color)
if __name__ == '__main__':
Example()
clr = JColorChooser()This code shows a color chooser dialog. The
color = clr.showDialog(self.panel, "Choose Color", Color.white)
self.display.setBackground(color)
showDialog()
method returns the selected color value. We change the display panel background to the newly selected color. 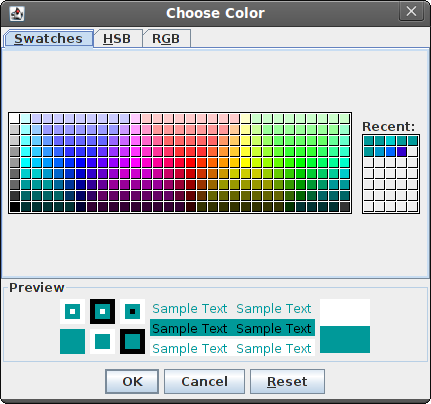
Figure: ColorDialog
JFileChooser
JFileChooser dialog allows user to select a file from the filesystem.#!/usr/local/bin/jythonIn our code example, we use the
# -*- coding: utf-8 -*-
"""
ZetCode Jython Swing tutorial
In this program, we use the
JFileChooser to select a file from
a filesystem.
author: Jan Bodnar
website: www.zetcode.com
last modified: November 2010
"""
from java.awt import BorderLayout
from javax.swing import BorderFactory
from javax.swing import JFileChooser
from javax.swing import JTextArea
from javax.swing import JScrollPane
from javax.swing import JButton
from javax.swing import JToolBar
from javax.swing import JPanel
from javax.swing import JFrame
from javax.swing.filechooser import FileNameExtensionFilter
class Example(JFrame):
def __init__(self):
super(Example, self).__init__()
self.initUI()
def initUI(self):
self.panel = JPanel()
self.panel.setLayout(BorderLayout())
toolbar = JToolBar()
openb = JButton("Choose file", actionPerformed=self.onClick)
toolbar.add(openb)
self.area = JTextArea()
self.area.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10))
pane = JScrollPane()
pane.getViewport().add(self.area)
self.panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10))
self.panel.add(pane)
self.add(self.panel)
self.add(toolbar, BorderLayout.NORTH)
self.setTitle("File chooser")
self.setSize(300, 250)
self.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
self.setLocationRelativeTo(None)
self.setVisible(True)
def onClick(self, e):
chooseFile = JFileChooser()
filter = FileNameExtensionFilter("c files", ["c"])
chooseFile.addChoosableFileFilter(filter)
ret = chooseFile.showDialog(self.panel, "Choose file")
if ret == JFileChooser.APPROVE_OPTION:
file = chooseFile.getSelectedFile()
text = self.readFile(file)
self.area.setText(text)
def readFile(self, file):
filename = file.getCanonicalPath()
f = open(filename, "r")
text = f.read()
return text
if __name__ == '__main__':
Example()
JFileChooser
dialog to select a C file and display its contents in a JTextArea
. self.area = JTextArea()This is the
JTextArea
in which we will show the contents of a selected file. chooseFile = JFileChooser()We create an instance of the
filter = FileNameExtensionFilter("c files", ["c"])
chooseFile.addChoosableFileFilter(filter)
JFileChooser
dialog. We create a filter which will show only C files. ret = chooseFile.showDialog(self.panel, "Choose file")The dialog is shown on the screen. We get the return value.
if ret == JFileChooser.APPROVE_OPTION:If the user has selected a file, we get the name of the file. Read its contents and set the text to the text area component.
file = chooseFile.getSelectedFile()
text = self.readFile(file)
self.area.setText(text)
def readFile(self, file):This code reads the text from the file. The
filename = file.getCanonicalPath()
f = open(filename, "r")
text = f.read()
return text
getCanonicalPath()
returns an absolute file name. 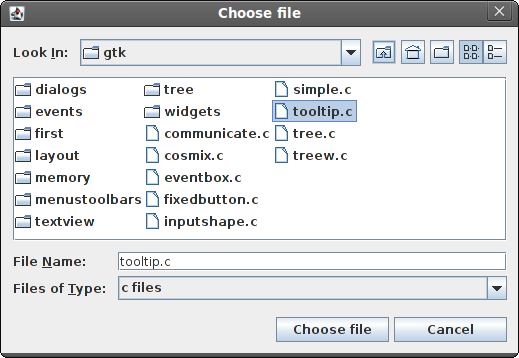
Figure: JFileChooser
In this part of the Jython Swing tutorial, we worked with dialog windows.
No comments:
Post a Comment